Interpolation
We know that the decorator functions of @Component take object and this object contains many properties. So we will learn about the interpolation properties in this article.
Before we get to know interpolation, we need to know the data Binding in Angular. Let's start with the data Binding.
The data binding is a powerful feature of Angular, that allows us to communicate between the component and its view. The data binding can be a one-way data binding [angular interpolation / string interpolation, linking properties, event linking] or a two-way data binding.
In the one-way data binding, the model value is inserted into an HTML element (DOM) and the model cannot be updated from the view. In the two-way binding, the automatic synchronization of data occurs between the Model and the View (each time the Model changes, it will be reflected in the View and vice versa)
Angular has four types of Data binding
- 1. Interpolation / String Interpolation (one-way data binding)
- 2. Property Binding (one-way data binding)
- 3. Event Binding (one-way data binding)
- 4. Two-Way Binding
Now we will learn the interpolation (one-way data binding)
Interpolation
Interpolation is a technique that allows the user to bind a value to an element of the user interface.
Interpolation binds data in one-way. This means that when you change the value of the bound field by interpolation, it is also updated on the page. The field value cannot be changed. An object of the component class is used as a data context for the component template. Therefore, the value to be bound in the view must be assigned to a field in the component class.
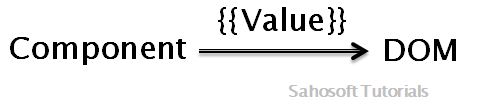
To interpolate a data, it must appear within an element of the user interface, such as {{city}}, or use parentheses.
Simple interpolation
The following code snippet shows an interpolation expression to bind the field city of the appComponent:
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` The city is: <strong> {{city}} </strong>` }) export class AppComponent { title = 'app'; city = 'delhi'; }
To see how it works, you can replace the appComponent template with the previous code snippet.
When the component is represented on a page with the previous code snippet in the template, print the value of the city field in the view. The below image shows the output of this snippet:
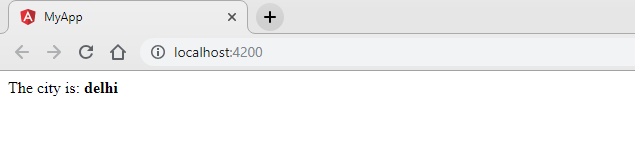
Modify value in interpolation
The syntax of binding a field using double brackets is called a link expression. Let's try to change the value of the city and see the user interface changes.
For this, edit the city value using button click. Add the following code snippet to the component :
After making this change, when the page is loaded, show the city as Noida.
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` The city is: <strong> {{city}} </strong>` }) export class AppComponent { title = 'app'; city = 'delhi'; }
The below image shows the output of this snippet:
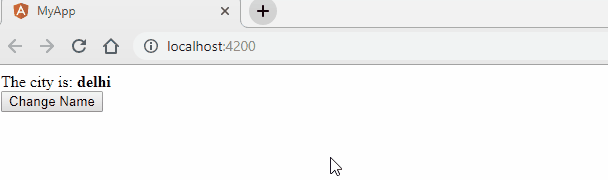
Concatenating fields in interpolation
We can concatenate a value from the static chain to the variable city value within the binding expression. The following code snippet greets the city with "hello":
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <div>{{'Hello, ' + city}}</div> ` }) export class AppComponent { title = 'app'; city = 'delhi'; }
The below image shows the output of this snippet:
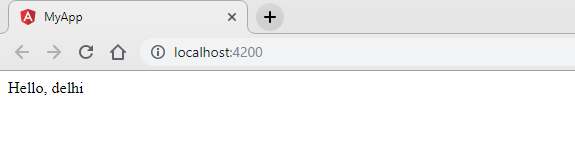
We can also concatenate several string fields in the expression. Concatenate the value of the position of the field and the value of the city in an expression. Modify the component template as follows:
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <div>{{greet + ',' + city}}</div> ` }) export class AppComponent { title = 'app'; city = 'delhi'; greet = 'Hello !'; }
The below image shows the output of this snippet:
Arithmetic operations in interpolation
Similar operations can also be performed using numeric fields. Add the following numeric fields to the component to explore its use:
We can directly show the values of these fields, perform simple arithmetic operations using fixed values or using the value of another field in a binding expression. The following code snippet shows some examples using the above fields:
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <div>{{num1+1}}</div> <div>{{num1+num2}}</div> <div>{{num1-num2}}</div> <div>{{num1*num2}}</div> <div>{{num2/num1}}</div> ` }) export class AppComponent { title = 'app'; num1: number = 10; num2: number = 100; }
The below image shows the output of this snippet:
bind property values into an object in interpolation
We can bind property values into an object using binding expressions. In the appcomponent created above, the field is assigned with an object. We will show the different properties of the object on the page. For this, we need to include the following piece of code in the controller template:
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <div>Name: {{company.name}}</div> <div>City: {{company.city}}</div> <div>State: {{company.state}}</div> <div>Country: {{company.country}}</div> ` }) export class AppComponent { title = 'app'; company = { name: 'Sahosoft Tutorials', city: 'Noida', state: 'UP', country: 'India' }; }
The below image shows the output of this snippet:
bind values using setTimeout function in interpolation
Values are not always available in the components. Sometimes, we may have to wait for an HTTP request to complete before assigning the value to the object. When the application waits for the HTTP request to complete, the above binding expressions will fail because the object value is not initially defined.
To simulate this behavior, assign a value to the position of the field after two seconds using a setTimeout function. This must be done in the component constructor.
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <div>Name: {{company.name}}</div> <div>City: {{company.city}}</div> <div>State: {{company.state}}</div> <div>Country: {{company.country}}</div> ` }) export class AppComponent { title = 'app'; company: any; constructor() { setTimeout(() => { this.company = { name: 'Sahosoft Tutorials', city: 'Noida', state: 'UP', country: 'India' }; }, (2000)); } }
The below image shows the output of this snippet:
Error handling while bind property values into an object in interpolation
Suppose we have company variable of any type without any property like name,city,state,country.
When you load the component on a page after this change, an error will be reported stating "Unable to read the ‘name’ property of undefined." Angular will not analyze the rest of the template after finding an error, so we will not see errors for other properties of the object.
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <div>Name: {{company.name}}</div> <div>City: {{company.city}}</div> <div>State: {{company.state}}</div> <div>Country: {{company.country}}</div> ` }) export class AppComponent { title = 'app'; company: any; }
The below image shows the output and error of this snippet:
To avoid this error, we can check if the object has a value and then connect it:
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <div>Name: {{company && company.name}}</div> <div>City: {{company && company.city}}</div> <div>State: {{company && company.state}}</div> <div>Country: {{company && company.country}}</div> ` }) export class AppComponent { title = 'app'; company: any; }
The below image shows the output of this snippet:
Although it solves the problem, verifying the presence of a property's value in an object makes the expression cumbersome and difficult to maintain.
A better way is to use the safe navigation operator (?.) To securely connect internal fields. The following code snippet uses the protected browsing property to link the city name:
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <div>Name: {{company?.name}}</div> <div>City: {{company?.city}}</div> <div>State: {{company?.state}}</div> <div>Country: {{company?.country}}</div> ` }) export class AppComponent { title = 'app'; company: any; }
The below image shows the output of this snippet:
Operations to be performed in interpolation
As we have seen, interpolation can be used to bind any value on the page. We can perform some simple operations within expressions to change values. But this does not mean that we can perform any JavaScript operation within the binding expressions.
The following types of JavaScript operations are not allowed in binding expressions:
- Assignments, increment (++) and decrement (--)
- Creation of objects
- Bitwise operators (| and &)
Because binding expressions are evaluated in each browser event to keep the view in synchronized with the data, they must be simple and independent. Mixing arithmetic expressions with interpolation could cause the following side effects:
- Increase the complexity of the markup.
- You can change the value of the field, which would add an inconsistency, since it changes the data in the same event cycle and, therefore, the changed value may not be displayed
- It also affects performance, since interpolation must be evaluated by the framework
- Because it is not the recommended way, a change in the frame to disable this function will interrupt the applications
Values bound within binding expressions are evaluated when a change in the status of the values occurs and if the expression triggers another change, it can enter an infinite loop. Therefore, keeping the simple expressions will reduce the time needed to evaluate the expressions and ultimately make the application work better.