template & templateUrl
We know that the @Component decorator functions take an object and this object contains many properties. So we will learn about the properties of the template & templateUrl in this article.
We can render our HTML code within the @Component decorator in two ways.
Inline Templates
The Inline templates are specified directly in the component decorator. In this, we will find the HTML inside the TypeScript file. This can be implemented using the "template" property.
The literal values of the template with back-ticks marks allow strings on multiple lines. It means that if you have HTML on more than one line, you should use backticks instead of single or double quotes, as shown below.
Use the following code in “app.component.ts” .
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', template: ` <H1>Template</H1> <hr> <div> Template inside typescript file </div>` }) export class AppComponent { title = 'app'; }
This will be the output of the above code.
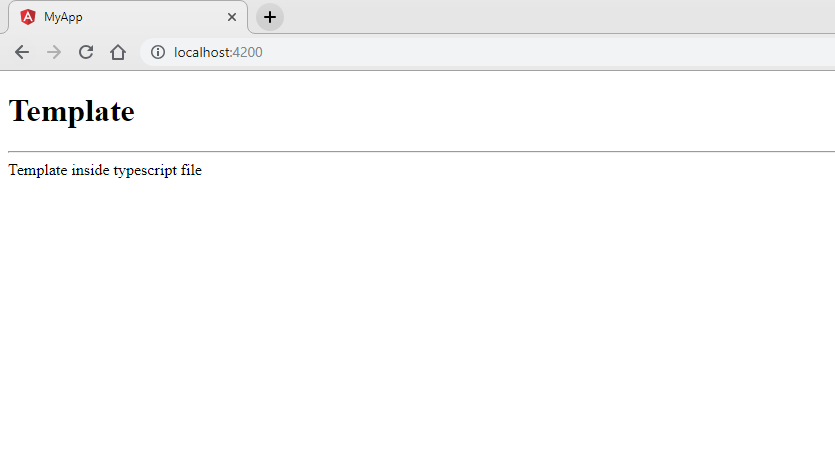
Note: that these elements cannot appear in a template: html, script, body and base.
External Template
The External templates define HTML in a separate file and refer to this file in templateUrl.means. In this, we will find a separate HTML file instead of finding the HTML inside the TS file. Here, the TypeScript file contains the path to that HTML file with the help of the "templateUrl" property.
Use the following code in “app.component.ts” file.
app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html' }) export class AppComponent { title = 'app'; }
Use the following code in “app.component.html” file.
app.component.html
<H1>templateUrl</H1> <hr> <div> Template outside typescript file. An implementation of templateUrl property. </div>
This will be the output of the above code.
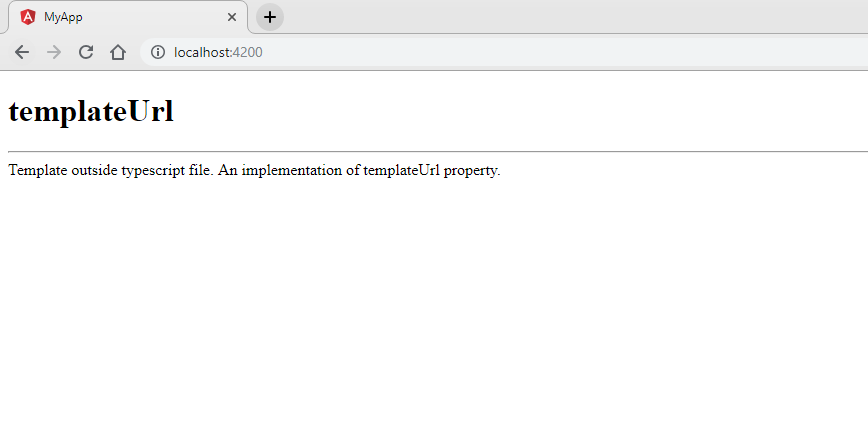
Needs to remember below points about template properties
• Each of these two must be presented in the component to link it to an external template (ie templateUrl) or an inline template (ie a template). Therefore, this is one of the properties you must have at all times.
• If we use the "template" and "templateUrl" both properties, it will generate errors
The official Angular Style Guide recommends extracting the templates into a separate file if the view template has more than 3 lines.
Let's try to understand why it is better to extract a view template in a separate file if it is longer than 3 lines.
With an inline template
1. We lose the features of the Visual Studio editor, like intellisense, code-completion and formatting.
2. TypeScript code is not easy to read and understand if combined with the HTML inline template .
3. The view template is inline in a pair of backtick marks. The question that comes to mind is why we cannot include HTML in a pair of single or double quotes. The answer is "YES", we can as long as the HTML is on a single line. This means that the above code can be rewritten using a pair of single quotes as shown below.
4. If the HTML is present in more than one line, backticks should be used instead of single or double quotation marks, as shown below. If you use single or double quotes instead of backticks, an error will occur.
template: “<h1>{{interpolation}}</h1>”, or template: ‘<h1>{{interpolation}}</h1>’,
With an external view template
1. We have the features of Visual Studio editor, intelligence and code -completion and formatting .
2. Not only is the code in "app.component.ts" is clean, it is also easier to read and understand.